Build 21228 has been released. Docker images available from DockerHub as usual, and bare-metal packages here.
Home Assistant up to version 2021.8.6 supported; the online version of the manual will now state the current supported versions; Fix an error in OWMWeatherController that could cause it to stop updating; Unify the approach to entity filtering on all hub interface classes (controllers); this works for device entities only; it may be extended to other entities later; Improve error detail in messages for EzloController during auth phase; Add isRuleSet() and isRuleEnabled() functions to expressions extensions; Implement set action for lock and passage capabilities (makes them more easily scriptable in some cases); Fix a place in the UI where 24-hour time was not being displayed.I need a handful of victims volunteers to help test previews of the next build of Reactor. A long-standing request was for "a simple login mechanism," but in practice, adding user authentication and competent access control turned out to be a pretty big project with a lot of big changes on both server and client sides. It's a bit more than I'm comfortable testing myself and springing out to everyone at once, so I'd like to work with a small group to put it through "sea trials."
Major changes/features include:
User authentication with hashed password storage; User group configuration with application restriction (admin, dashboard, API); Detailed control over API access, with user- and token-based authentication/authorization; Improvements to the HTTPS service; Improvements to UI coordination with the core for Rules and Reactions.If this sounds like something you'd like to help with, drop me a reply here in this thread or privately.
@toggledbits I have finally finished up the SSL using Let's Encrypt and am getting this from my local browser:
f3d0ac22-272e-46c1-b7e3-57b08bdd1555-image.png
21c04fe1-1760-4ce6-a4de-2285d3349940-image.png
3a7022db-5add-40a1-b9a2-0c0b97fa211b-image.png
I know you said in the docs that using a self-signed could lead to this but this is LE.
Hi @toggledbits,
I don't know if I'm the only one, so I'm reporting here first instead of opening a bug.
Basically, with the latest 2-3 updates of Reactor and MQTTController, after a restart previous statuses are lost (for both Virtual and MQTT entities), until they're restored.
It's particularly annoying for Virtual Entities, because I have to set them all over again (I've coded some defaults at startup if the values are empty, but sometimes these are not the correct values before the update).
Not easy to reproduce, and logs are gone, but the first time I tought it was me hallucinating, the second one didn't bother too much, after the third I realized it's something not coming from me.
the behavior could be seen in this screenshot:
1c007fc2-4dbc-4476-8dca-e5aa111e4642-image.png
Any hint is appreciated.
I'm slowly migrating all my stuff to MQTT under MSR, so I have a central place to integrate everything (and, in a not-so-distant future, to remove virtual devices from my Vera and leave it running zwave only).
Anyway, here's my reactor-mqtt-contrib package:
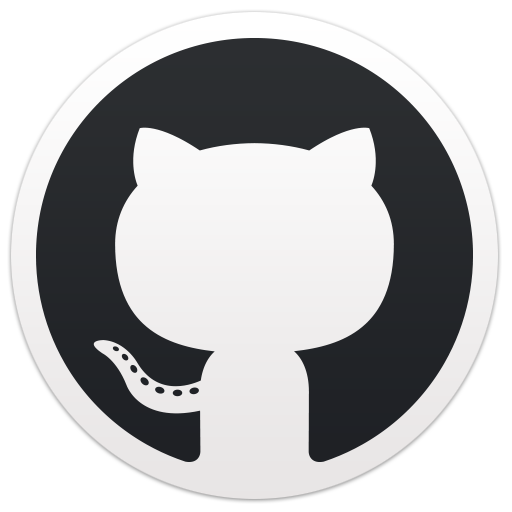
Contrib MQTT templates for Reactor. Contribute to dbochicchio/reactor-mqtt-contrib development by creating an account on GitHub.
Simply download yaml files (everything or just the ones you need) and you're good to go.
I have mapped my most useful devices, but I'll add others soon. Feel free to ask for specific templates, since I've worked a lot in the last weeks to understand and operate them.
The templates are supporting both init and query, so you have always up-to-date devices at startup, and the ability to poll them. Online status is supported as well, so you can get disconnected devices with a simple expression.
Many-many thanks to @toggledbits for its dedication, support, and patience with me and my requests 🙂
Good morning,
So Home Assistant decided to change the default weather home format that I've been using for the past year and a half. I had two Global Expressions set up to pull the high and low temp forecast for the day. Now it's pulling null values.
094c9205-cc9e-4fcc-ac4f-1bf54acea299-image.png
In the dev tools, it now uses a new service (Weather. get forecasts), plural, where the old Weather.get forecast is depreciated and now longer functions.
8c7a1fcc-dd3f-4268-a0b7-29d542f86adc-image.png
It shows a templow field, and a temperature field, which I presume is the forecast high.
When I head back over to MSR, I'm having a hard time finding those values in the Entities tab.
c5ea1048-a72e-4647-9c50-9d0c5fd20767-image.png
wx.asoftime=null wx.ceiling=null wx.ceiling_unit=null wx.cloud_cover=null wx.condition_code=null wx.description="partlycloudy" wx.feels_like=null wx.humidity=57 wx.humidity_unit="%" wx.icon=null wx.location=null wx.precipitation_1hr=null wx.precipitation_24hr=null wx.precipitation_other=null wx.precipitation_type=null wx.precipitation_unit="in" wx.pressure=30 wx.pressure_unit="inHg" wx.temperature=55 wx.temperature_unit="°F" wx.visibility=null wx.visibility_unit="mi" wx.wind_compass=210.3 wx.wind_conditions=null wx.wind_direction="SSW" wx.wind_gust=null wx.wind_speed=6.28 wx.wind_speed_unit="mph" x_hass.domain="weather" x_hass.entity_id="weather.forecast_home" x_hass.services=["weather"] x_hass.state="partlycloudy" x_hass_attr.attribution="Weather forecast from met.no, delivered by the Norwegian Meteorological Institute." x_hass_attr.cloud_coverage=85.9 x_hass_attr.dew_point=40 x_hass_attr.friendly_name="New Windsor Weather" x_hass_attr.humidity=57 x_hass_attr.precipitation_unit="in" x_hass_attr.pressure=30 x_hass_attr.pressure_unit="inHg" x_hass_attr.supported_features=3 x_hass_attr.temperature=55 x_hass_attr.temperature_unit="°F" x_hass_attr.visibility_unit="mi" x_hass_attr.wind_bearing=210.3 x_hass_attr.wind_speed=6.28 x_hass_attr.wind_speed_unit="mph"There is a x_hass_attr.temperature, but that appears to be the current temperature, not the high that I found on the dev tools screenshot.
Any ideas?
Running:
Core
2024.4.3
Supervisor
2024.04.0
Operating System
12.2
Frontend
20240404.2
MSR: latest-24057-e9add9f5
Hey Patrick, I recently have been noticing that MSR has been acting up ie. it's been needing restarts and has been slow. I began trouble shooting by looking at the logs and have noticed the following errors for a lot of entities. I thought maybe a simple reboot of RPi was needed and I kept seeing the same errors in the system logs. I am oddly enough not seeing these same errors in the MSR logs. Where things started getting weird is whenever I rebooted MSR it wouldn't come back online .I would have to restart the RPi then it would come back online. I just restarted MSR again to capture logs and it restarted fine, so I guess its good for now? I think this is more or so a corrupted SD card issue rather a MSR issue but well being troubleshooting from here. The SD card is about 1-2 years old.
Apologies if this post is everywhere, I cannot consistently recreate any oddities that are happening, that's what is leading me to believe my SD is going bad.
PS: If anyone knows how to diagnose a corrupt SD card please chime in.
MSR latest-24057-e9add9f5
Home Assistant 2024.4.3
Raspberry Pi 3b+
This system has been running flawlessly year after year for the time changes twice a year literally since MSR came out so I was caught off-guard when this happened this morning.
Time in MSR browser is EST, time on RPi is local time (DST).
76ed5313-b9b9-46d4-b0f9-462c40e99750-image.png
195e61c5-58a7-4453-b96a-18cebae75550-image.png
I've rebooted the RPi I've restarted MSR after double-checking the time on the RPi. Used a completely different browser to eliminate any caching concerns. Double-checked MSR reactor.yamla5f23151-d691-4343-8499-8e77a55528e5-image.png
What am I missing here @toggledbits ?
Hi,
For the standard capabilities MSR sends both a value record and a units record to InfluxDB. The latter I would like not to send as they are not really any use for me and it will reduce the number of records send to my InfluxDB.
Is there a quick way to do this with a filter_entities line like: *>units?
Or do I have to update all capabilities to read like this:
power_sensor:
attributes:
value: true
Cheers Rene
I'm trying to replicate this
wallbox_set_number.PNG
into a MQTT entity where I could set a number with a min and max value.
I can't find a standard capability that fits or any documentation on local MQTT capabilities and the only post on the forum mentioning local MQTT capabilities is this post, is it even possible in current release?
My trial and error work in local_mqtt_capabilities.yaml isn't much to show as it's just a copy of mqtt_capabilities.yaml with changed names and then I got stuck.
Any guidance, examples, documentation, future feature request or denial would be much appreciated, thanks!
Reactor 24057-e9add9f5 bare metal
MQTTController 24050
Hi guys,
I've recently bought a new Govee outdoor permanent lights set, and I love it. WAF is pretty high, and the product is good quality. I hope to never run lights in the front of the house.
This new addition has found me searching for something to control these lights, locally. Govee has officials remote and LAN APIs and Home Assistant has it supported, but some undocumented stuff that's integrated into an Homebridge plugin that seems very promising. Without this plugin, my playlist is orchestrated via the cloud and that makes zero sense.
In the past I got some inspiration from plugins running on other platforms and Homebridge seems one of the most active. I could map its devices via HomeKit-local on HA, but I've decommissioned Homebridge years ago when we settled to Alexa (and I want to stay simple), so I had an idea: why get inspiration and rewrite things, when you could write an Homebridge adapter that could load any Homebridge plugin and run them natively under Reactor (MSR)?
I'm not sure if that's viable or made any sense, so I'm posting here to get feedback, encouragement and your thoughts. Anyone could be potentially interested in such a thing?
Hi- looking for a hint in where to start. My goal is to set a PIN code in a zwave kwikset lock triggered in a rule.
The device isn’t exposing methods to help. The x-hass.call-service looks promising, but what would the service name be?
Plan b would be send the zwave controller a config command- I don’t see any way to explicitly send a command through JS Zwave in my environment.
Running reactor bare metal. JS Zwave is running as an add on inside HASS OS.
Any tips are appreciated.
Hey crew, I'm trying to use MSR to control the RGB values of a Z-Wave bulb in Home Assistant.
Problem I'm running into - I would like to use 'rgb_color.set' to control this, but it doesn't work, instead it always passes the values '255,255,255' to HA no matter what values I enter within MSR.
More notes and examples below - I'm wondering if this is a formatting issue that I'm missing? Thanks for any help!
NOTES FROM TROUBLESHOOTING:
'rgb_color.set_rgb' works successfully, which seems strange. You'd think they would both be affected I've tried a couple different formats, like adding quotes, adding/removing spaces between the RGB values, nothing has fixed it.EXAMPLES:
When I use 'rgb_color.set_rgb', the values successfully carry over to Home Assistant:
f0f4befc-a642-428e-8923-e5f856ca7e2b-image.png
0af0a4f8-50b9-4100-b1e8-52a0de4cbcbb-image.png
But when I use 'rgb_color.set', the values DO NOT successfully carry over to Home Assistant:
9e2d7004-8085-4b70-bb3e-45614b7260a0-image.png 0d630228-c74b-4db8-89bd-2572a08608a3-image.png
DETAILS:
Bulb is LZW42 by Inovelli MSR version: stable-23242-5ee8e1d4HA DETAILS
Core 2024.2.5 Supervisor 2024.02.1 Operating System 12.0Hi,
I’m running MSR in a docker container on my Synology Nas. The container is automatically updated using watchtower weekly.
It was working. Now, after the update, Reactor webpage is able to load, and all indications on the webpage suggests that it is working fine. However, the updated statuses from Home Assistant and Vera are not being detected.
The container logs show the following error
Reactor stable-23344-5aad7754 app 23344 configuration from /var/reactor/config NODE_PATH /opt/reactor:/opt/reactor/node_modules [stable-23344]2024-02-28T21:57:49.516Z <app:null> Reactor build stable-23344-5aad7754 starting on v16.15.1 [stable-23344]2024-02-28T21:57:49.517Z <app:null> Process ID 1 user/group 0/0; docker; platform linux/x64 #69057 SMP Fri Jan 12 17:02:28 CST 2024; locale (undefined) [stable-23344]2024-02-28T21:57:49.517Z <app:null> Basedir /opt/reactor; data in /var/reactor/storage [stable-23344]2024-02-28T21:57:49.517Z <app:null> NODE_PATH=/opt/reactor:/opt/reactor/node_modules [stable-23344]2024-02-28T21:57:49.696Z <Structure:null> Module Structure v23172 [stable-23344]2024-02-28T21:57:49.698Z <Capabilities:null> Module Capabilities v23331 [stable-23344]2024-02-28T21:57:49.780Z <Plugin:null> Module Plugin v22300 [stable-23344]2024-02-28T21:57:49.790Z <TimerBroker:null> Module TimerBroker v22283 [stable-23344]2024-02-28T21:57:49.794Z <Entity:null> Module Entity v22353 [stable-23344]2024-02-28T21:57:49.866Z <Controller:null> Module Controller v23069 [stable-23344]2024-02-28T21:57:50.030Z <default:null> Module Ruleset v22293 [stable-23344]2024-02-28T21:57:50.031Z <default:null> Module Rulesets v22146 [stable-23344]2024-02-28T21:57:50.066Z <GlobalExpression:null> Module GlobalExpression v23211 [stable-23344]2024-02-28T21:57:50.581Z <Predicate:null> Module Predicate v23093 [stable-23344]2024-02-28T21:57:50.595Z <AlertManager:null> Module AlertManager v22283 [stable-23344]2024-02-28T21:57:50.600Z <Rule:null> Module Rule v23107 [stable-23344]2024-02-28T21:57:50.614Z <GlobalReaction:null> Module GlobalReaction v22324 [stable-23344]2024-02-28T21:57:50.617Z <Engine:null> Module Engine v23339 [stable-23344]2024-02-28T21:57:50.635Z <httpapi:null> Module httpapi v23058 [stable-23344]2024-02-28T21:57:50.680Z <wsapi:null> Module wsapi v23172 [stable-23344]2024-02-28T21:57:50.789Z <TaskQueue:null> Module TaskQueue 21351 [stable-23344]2024-02-28T21:57:50.790Z <VeraController:null> Module VeraController v23109 [stable-23344]2024-02-28T21:57:50.971Z <HassController:null> Module HassController v23344 [stable-23344]2024-02-28T21:57:51.716Z <DynamicGroupController:null> Module DynamicGroupController v22313 [stable-23344]2024-02-28T21:57:52.253Z <SystemController:null> Module SystemController v23331 i18n: missing en-US language string: The version of nodejs you are using ({0}) is now end-of-life, and so is deprecated for use with Reactor. Please upgrade nodejs to {2}.{3} or higher as soon as possible; the current LTS version is recommended. Releases of Reactor produced after {1} will not run under this version of nodejs at all. [stable-23344]2024-02-28T21:57:52.256Z <Controller:CRIT> SyntaxError: Unexpected end of JSON input [-] SyntaxError: Unexpected end of JSON input at JSON.parse (<anonymous>) at /opt/reactor/server/lib/Controller.js:464:51 at Array.forEach (<anonymous>) at SystemController._restoreEntities (/opt/reactor/server/lib/Controller.js:458:36) at new Controller (/opt/reactor/server/lib/Controller.js:45:42) at new SystemController (/opt/reactor/server/lib/SystemController.js:29:9) at /opt/reactor/server/lib/Controller.js:101:37 Trace: The version of nodejs you are using ({0}) is now end-of-life, and so is deprecated for use with Reactor. Please upgrade nodejs to {2}.{3} or higher as soon as possible; the current LTS version is recommended. Releases of Reactor produced after {1} will not run under this version of nodejs at all. at _T (/opt/reactor/server/lib/i18n.js:468:37) at AlertManager.addAlert (/opt/reactor/server/lib/AlertManager.js:126:25) at /opt/reactor/app.js:381:140 [stable-23344]2024-02-28T21:57:59.313Z <app:CRIT> SyntaxError: Unexpected end of JSON input [-] SyntaxError: Unexpected end of JSON input at JSON.parse (<anonymous>) at IndividualFileStrategy.getDataObject (/opt/reactor/server/lib/IndividualFileStrategy.js:114:54) at DelayWriteCacheStrategy.getDataObject (/opt/reactor/server/lib/DelayWriteCacheStrategy.js:87:50) at Container.getDataObject (/opt/reactor/server/lib/Container.js:69:67) at Function.getInstance (/opt/reactor/server/lib/Data.js:37:179) at Rule.getRuleStates (/opt/reactor/server/lib/Rule.js:507:100) at Rule.getConditionState (/opt/reactor/server/lib/Rule.js:538:47) at new Rule (/opt/reactor/server/lib/Rule.js:378:47) at Function.getInstance (/opt/reactor/server/lib/Rule.js:387:36) at /opt/reactor/server/lib/Engine.js:263:53 i18n: missing en-US language string: HomeAssistant on {0:q} may be an unsupported version. The reported version ({1}) has not been certified/tested with this version of Reactor and may cause errors. You must either modify your HomeAssistant install, or see if an update to Reactor has been made available. Trace: HomeAssistant on {0:q} may be an unsupported version. The reported version ({1}) has not been certified/tested with this version of Reactor and may cause errors. You must either modify your HomeAssistant install, or see if an update to Reactor has been made available. at _T (/opt/reactor/server/lib/i18n.js:468:37) at AlertManager.addAlert (/opt/reactor/server/lib/AlertManager.js:126:25) at HassController.sendWarning (/opt/reactor/server/lib/Controller.js:197:36) at /opt/reactor/server/lib/HassController.js:1117:370 at processTicksAndRejections (node:internal/process/task_queues:96:5)I’ve tried using “latest-amd64” and it does not work either. The logs show similar json input error.
Reactor latest-24057-e9add9f5 app 24052 configuration from /var/reactor/config NODE_PATH /opt/reactor:/opt/reactor/node_modules [latest-24057]2024-02-28T22:27:30.466Z <app:null> Reactor build latest-24057-e9add9f5 starting on v20.10.0 [latest-24057]2024-02-28T22:27:30.522Z <app:null> Process ID 1 user/group 0/0; docker; platform linux/x64 #69057 SMP Fri Jan 12 17:02:28 CST 2024; locale (undefined) [latest-24057]2024-02-28T22:27:30.522Z <app:null> Basedir /opt/reactor; data in /var/reactor/storage [latest-24057]2024-02-28T22:27:30.522Z <app:null> NODE_PATH=/opt/reactor:/opt/reactor/node_modules [latest-24057]2024-02-28T22:27:30.667Z <Structure:null> Module Structure v23172 [latest-24057]2024-02-28T22:27:30.673Z <Capabilities:null> Module Capabilities v23331 [latest-24057]2024-02-28T22:27:30.780Z <Plugin:null> Module Plugin v22300 [latest-24057]2024-02-28T22:27:30.787Z <TimerBroker:null> Module TimerBroker v22283 [latest-24057]2024-02-28T22:27:30.791Z <Entity:null> Module Entity v22353 [latest-24057]2024-02-28T22:27:30.796Z <Controller:null> Module Controller v23069 [latest-24057]2024-02-28T22:27:30.811Z <default:null> Module Ruleset v22293 [latest-24057]2024-02-28T22:27:30.811Z <default:null> Module Rulesets v22146 [latest-24057]2024-02-28T22:27:30.821Z <GlobalExpression:null> Module GlobalExpression v23211 [latest-24057]2024-02-28T22:27:30.890Z <Predicate:null> Module Predicate v23093 [latest-24057]2024-02-28T22:27:30.958Z <AlertManager:null> Module AlertManager v22283 [latest-24057]2024-02-28T22:27:31.027Z <Rule:null> Module Rule v24057 [latest-24057]2024-02-28T22:27:31.033Z <GlobalReaction:null> Module GlobalReaction v22324 [latest-24057]2024-02-28T22:27:31.036Z <Engine:null> Module Engine v24023 [latest-24057]2024-02-28T22:27:31.042Z <httpapi:null> Module httpapi v24057 [latest-24057]2024-02-28T22:27:31.216Z <wsapi:null> Module wsapi v24057 [latest-24057]2024-02-28T22:27:31.296Z <TaskQueue:null> Module TaskQueue 21351 [latest-24057]2024-02-28T22:27:31.297Z <VeraController:null> Module VeraController v24050 [latest-24057]2024-02-28T22:27:31.365Z <HassController:null> Module HassController v24048 [latest-24057]2024-02-28T22:27:31.659Z <DynamicGroupController:null> Module DynamicGroupController v22313 [latest-24057]2024-02-28T22:27:31.668Z <SystemController:null> Module SystemController v23331 [latest-24057]2024-02-28T22:27:31.673Z <Controller:CRIT> SyntaxError: Unexpected end of JSON input [-] SyntaxError: Unexpected end of JSON input at JSON.parse (<anonymous>) at /opt/reactor/server/lib/Controller.js:464:51 at Array.forEach (<anonymous>) at SystemController._restoreEntities (/opt/reactor/server/lib/Controller.js:458:36) at new Controller (/opt/reactor/server/lib/Controller.js:45:43) at new SystemController (/opt/reactor/server/lib/SystemController.js:237:9) at /opt/reactor/server/lib/Controller.js:101:37 [latest-24057]2024-02-28T22:27:38.845Z <app:CRIT> SyntaxError: Unexpected end of JSON input [-] SyntaxError: Unexpected end of JSON input at JSON.parse (<anonymous>) at IndividualFileStrategy.getDataObject (/opt/reactor/server/lib/IndividualFileStrategy.js:51:45) at DelayWriteCacheStrategy.getDataObject (/opt/reactor/server/lib/DelayWriteCacheStrategy.js:89:49) at Container.getDataObject (/opt/reactor/server/lib/Container.js:69:65) at Data.getInstance (/opt/reactor/server/lib/Data.js:45:179) at Rule.getRuleStates (/opt/reactor/server/lib/Rule.js:515:101) at Rule.getConditionState (/opt/reactor/server/lib/Rule.js:546:47) at new Rule (/opt/reactor/server/lib/Rule.js:371:47) at Rule.getInstance (/opt/reactor/server/lib/Rule.js:380:36) at /opt/reactor/server/lib/Engine.js:828:53 i18n: missing en-US language string: HomeAssistant on {0:q} may be an unsupported version. The reported version ({1}) has not been certified/tested with this version of Reactor and may cause errors. You must either modify your HomeAssistant install, or see if an update to Reactor has been made available. Trace: HomeAssistant on {0:q} may be an unsupported version. The reported version ({1}) has not been certified/tested with this version of Reactor and may cause errors. You must either modify your HomeAssistant install, or see if an update to Reactor has been made available. at _T (/opt/reactor/server/lib/i18n.js:614:37) at AlertManager.addAlert (/opt/reactor/server/lib/AlertManager.js:128:25) at HassController.sendWarning (/opt/reactor/server/lib/Controller.js:197:36) at /opt/reactor/server/lib/HassController.js:1133:374 at process.processTicksAndRejections (node:internal/process/task_queues:95:5)How do I fix this?
Noticed right away last night at closing time that the open/close had become inverted with the update to v23326.
It wasn't an awful bit of lift to change all Reactions to reflect the change but it was jarring initially when everything flipped.
Are there release notes on that version, @toggledbits ?
Installing latest-24052-d039d560 and I did a dumb thing: moving too quickly, I deleted package.json instead of package-lock.json because the latter didn't exist.
Ran the installer script - but in the wrong directory so I moved package.json manually.
Everything has come back EXCEPT for my MQTT Cloud OwnTracks entities. They're erroring because of this:
eb6f35dc-1981-432a-993d-aefae54cba02-image.png
(Don't heckle my folder structure - it's run this way for three years)
reactor.yaml is fine, all MQTT configs exactly as they should be.
The folder mqtt_templates is present:
f57fa07d-516b-4c8e-8cb0-eeeeaf34de14-image.png
The file it says is "unreadable" is present and hasn't changed (today's date is the Changed date because I did a quick nonsense edit to see that it was not corrupted in some way).
b8fc46a1-f66f-417d-b095-c8e3744ccbe8-image.png
The logs show:
[latest-24052]2024-02-22T01:04:40.320Z <ZWaveJSController:WARN> zwavejs_capabilities defines x_zwave_device_cfg, which is not consistent with the recommended naming [latest-24052]2024-02-22T01:04:40.321Z <ZWaveJSController:WARN> zwavejs_capabilities defines x_zwave_mfg_spec, which is not consistent with the recommended naming [latest-24052]2024-02-22T01:04:40.321Z <ZWaveJSController:WARN> zwavejs_capabilities defines x_zwave_device_ver, which is not consistent with the recommended naming [latest-24052]2024-02-22T01:04:40.322Z <ZWaveJSController:WARN> zwavejs_capabilities defines x_zwave_values, which is not consistent with the recommended naming [latest-24052]2024-02-22T01:04:40.323Z <Controller:INFO> ZWaveJSController#zwavejs loaded zwavejs capabilities ver 23194 rev 1 format 1 [latest-24052]2024-02-22T01:04:40.338Z <Controller:INFO> ZWaveJSController#zwavejs loaded implementation data ver 23254 rev 1 format 1 [latest-24052]2024-02-22T01:04:40.339Z <Structure:INFO> Starting controller SystemController#reactor_system [latest-24052]2024-02-22T01:04:40.345Z <Controller:NOTICE> Controller SystemController#reactor_system is now online. [latest-24052]2024-02-22T01:04:40.572Z <HassController:INFO> HassController#hass device mapping data loaded; checking... [latest-24052]2024-02-22T01:04:40.574Z <HassController:WARN> HassController: implementation of capability battery_maintenance does not provide attribute state [latest-24052]2024-02-22T01:04:40.577Z <HassController:WARN> HassController: implementation of capability input_select.selector does not provide attribute values [latest-24052]2024-02-22T01:04:40.586Z <MQTTController:ERR> MQTTController#mqtt can't read/load templates from /home/reactor/Documents/reactor/config/mqtt_templates/owntracks_sensor.yaml: [Error] Function yaml.safeLoad is removed in js-yaml 4. Use yaml.load instead, which is now safe by default. [-] [latest-24052]2024-02-22T01:04:40.595Z <MQTTController:CRIT> Error: Function yaml.safeLoad is removed in js-yaml 4. Use yaml.load instead, which is now safe by default. [-] Error: Function yaml.safeLoad is removed in js-yaml 4. Use yaml.load instead, which is now safe by default. at Object.safeLoad (/home/reactor/Documents/reactor/node_modules/js-yaml/index.js:10:11) at MQTTController._load_implementation (/home/reactor/Documents/reactor/ext/MQTTController/MQTTController.js:807:38) at async MQTTController.start (/home/reactor/Documents/reactor/ext/MQTTController/MQTTController.js:95:13) at async Promise.allSettled (index 3) [latest-24052]2024-02-22T01:04:40.597Z <MQTTController:ERR> MQTTController#mqtt2 can't read/load templates from /home/reactor/Documents/reactor/config/mqtt_templates/owntracks_sensor.yaml: [Error] Function yaml.safeLoad is removed in js-yaml 4. Use yaml.load instead, which is now safe by default. [-] [latest-24052]2024-02-22T01:04:40.603Z <MQTTController:CRIT> Error: Function yaml.safeLoad is removed in js-yaml 4. Use yaml.load instead, which is now safe by default. [-] Error: Function yaml.safeLoad is removed in js-yaml 4. Use yaml.load instead, which is now safe by default. at Object.safeLoad (/home/reactor/Documents/reactor/node_modules/js-yaml/index.js:10:11) at MQTTController._load_implementation (/home/reactor/Documents/reactor/ext/MQTTController/MQTTController.js:807:38) at async MQTTController.start (/home/reactor/Documents/reactor/ext/MQTTController/MQTTController.js:95:13) at async Promise.allSettled (index 4) [latest-24052]2024-02-22T01:04:40.608Z <HassController:NOTICE> HassController#hass connecting to ws://192.168.1.198:8123/api/websocket [latest-24052]2024-02-22T01:04:40.616Z <MQTTController:INFO> MQTTController#mqtt instance topic ident is mqtt [latest-24052]2024-02-22T01:04:40.617Z <Controller:INFO> MQTTController#mqtt 0 dead entities older than 86400000s purged [latest-24052]2024-02-22T01:04:40.619Z <MQTTController:NOTICE> MQTTController#mqtt connecting to broker at mqtt://192.168.1.198:1883/ [latest-24052]2024-02-22T01:04:40.691Z <MQTTController:INFO> MQTTController#mqtt2 instance topic ident is mqtt2 [latest-24052]2024-02-22T01:04:40.692Z <MQTTController:ERR> MQTTController#mqtt2 entity owntracks_iphone14p uses_template=owntracks_sensor, template not defined [latest-24052]2024-02-22T01:04:40.697Z <MQTTController:ERR> MQTTController#mqtt2 entity owntracks_iphone8 uses_template=owntracks_sensor, template not defined [latest-24052]2024-02-22T01:04:40.726Z <MQTTController:ERR> MQTTController#mqtt2 entity owntracks_iphone14jay uses_template=owntracks_sensor, template not defined [latest-24052]2024-02-22T01:04:40.730Z <MQTTController:ERR> MQTTController#mqtt2 entity owntracks_iphone14jen uses_template=owntracks_sensor, template not defined [latest-24052]2024-02-22T01:04:40.735Z <MQTTController:ERR> MQTTController#mqtt2 entity owntracks_iphone14addie uses_template=owntracks_sensor, template not defined [latest-24052]2024-02-22T01:04:40.768Z <MQTTController:ERR> MQTTController#mqtt2 entity owntracks_iphone14bella uses_template=owntracks_sensor, template not defined [latest-24052]2024-02-22T01:04:40.773Z <MQTTController:ERR> MQTTController#mqtt2 entity owntracks_androidaa uses_template=owntracks_sensor, template not defined [latest-24052]2024-02-22T01:04:40.777Z <MQTTController:NOTICE> MQTTController#mqtt2 device owntracks_androidaa no longer available, marking BinarySensor#mqtt2>owntracks_androidaa for removal [latest-24052]2024-02-22T01:04:40.781Z <MQTTController:NOTICE> MQTTController#mqtt2 device owntracks_iphone14addie no longer available, marking BinarySensor#mqtt2>owntracks_iphone14addie for removal [latest-24052]2024-02-22T01:04:40.786Z <MQTTController:NOTICE> MQTTController#mqtt2 device owntracks_iphone14bella no longer available, marking BinarySensor#mqtt2>owntracks_iphone14bella for removal [latest-24052]2024-02-22T01:04:40.790Z <MQTTController:NOTICE> MQTTController#mqtt2 device owntracks_iphone14jay no longer available, marking BinarySensor#mqtt2>owntracks_iphone14jay for removal [latest-24052]2024-02-22T01:04:40.794Z <MQTTController:NOTICE> MQTTController#mqtt2 device owntracks_iphone14jen no longer available, marking BinarySensor#mqtt2>owntracks_iphone14jen for removal [latest-24052]2024-02-22T01:04:40.798Z <MQTTController:NOTICE> MQTTController#mqtt2 device owntracks_iphone14p no longer available, marking BinarySensor#mqtt2>owntracks_iphone14p for removal [latest-24052]2024-02-22T01:04:40.802Z <MQTTController:NOTICE> MQTTController#mqtt2 device owntracks_iphone8 no longer available, marking BinarySensor#mqtt2>owntracks_iphone8 for removal [latest-24052]2024-02-22T01:04:40.807Z <Controller:INFO> MQTTController#mqtt2 0 dead entities older than 86400000s purged [latest-24052]2024-02-22T01:04:40.808Z <MQTTController:NOTICE> MQTTController#mqtt2 connecting to broker at mqtts://7ef71f0117fe40d28c2df12205c4a0d0.s2.eu.hivemq.cloud:8883 [latest-24052]2024-02-22T01:04:40.816Z <VirtualEntityController:INFO> VirtualEntityController#virtual configuring virtual Driving Flag - 2 (virt1) [latest-24052]2024-02-22T01:04:40.826Z <VirtualEntityController:INFO> VirtualEntityController#virtual configuring virtual Far Away Flag - 2 (virt2) [latest-24052]2024-02-22T01:04:40.827Z <VirtualEntityController:INFO> VirtualEntityController#virtual configuring virtual Garage Door Momentary Contact vSwitch (virt3) [latest-24052]2024-02-22T01:04:40.828Z <Controller:INFO> VirtualEntityController#virtual 0 dead entities older than 86400000s purged [latest-24052]2024-02-22T01:04:40.829Z <ZWaveJSController:INFO> ZWaveJSController#zwavejs connecting to ws://192.168.1.198:3000 [latest-24052]2024-02-22T01:04:40.836Z <Controller:NOTICE> Controller VirtualEntityController#virtual is now online. [latest-24052]2024-02-22T01:04:40.839Z <app:NOTICE> Starting HTTP server and API... [latest-24052]2024-02-22T01:04:40.845Z <httpapi:NOTICE> httpapi: starting HTTP service on port 8111 [latest-24052]2024-02-22T01:04:40.854Z <app:NOTICE> Starting Reaction Engine... [latest-24052]2024-02-22T01:04:40.856Z <Engine:INFO> Reaction Engine starting [latest-24052]2024-02-22T01:04:40.857Z <Engine:INFO> Checking rule sets... [latest-24052]2024-02-22T01:04:40.880Z <Engine:INFO> Checking rules... [latest-24052]2024-02-22T01:04:41.421Z <Engine:INFO> Data check complete; no corrections. [latest-24052]2024-02-22T01:04:41.571Z <Rule:NOTICE> Driving Flag (rule-l97r2umw in Shared Rules) starting [latest-24052]2024-02-22T01:04:41.572Z <Rule:NOTICE> Driving Flag - Far Away (rule-l97r814k in Shared Rules) starting [latest-24052]2024-02-22T01:04:41.573Z <Rule:NOTICE> DST (rule-l118lzpd in Shared Rules) starting [latest-24052]2024-02-22T01:04:41.573Z <Rule:NOTICE> HVAC Cooling Master (Local APIs) (rule-ktinsq18 in Shared Rules) starting [latest-24052]2024-02-22T01:04:41.574Z <Rule:NOTICE> HVAC Heating before Alarm times (rule-l7hirm3d in Shared Rules) starting [latest-24052]2024-02-22T01:04:41.574Z <Rule:NOTICE> HVAC Heating Master (Local APIs) (rule-ktio3vc1 in Shared Rules) starting [latest-24052]2024-02-22T01:04:41.575Z <Rule:NOTICE> HVAC Neutral Master (rule-ktint8c3 in Shared Rules) starting [latest-24052]2024-02-22T01:04:41.575Z <Rule:NOTICE> It's Raining (rule-lfs6r1lq in Shared Rules) starting [latest-24I'm flummoxed.
I was using this method here by @therealdb to run Lua Code from MSR on my Vera Plus but it seems to have stopped working now.
Its the one where you uploaded a file called VeraScenes.lua to your Vera hub and then you could call functions of that file to run particular bits of Lua code from an MSR reaction etc.
9233b0a7-ec07-4ae5-99c0-cdf9c709d57c-image.png
This was working well for a long time but I noticed today its not working now.
Looking in the MSR log after I ran a reaction in one of my rules I just saw this:
[latest-23338]2024-02-19T18:41:35.650Z <Engine:INFO> Enqueueing "Front Cam Motion Detection ON or OFF Old Method<RESET>" (rule-klten9w9:R) [latest-23338]2024-02-19T18:41:35.653Z <Engine:5:Engine.js:1466> [Engine]Engine#1 enqueued reaction rule-klten9w9:R as 22989 [latest-23338]2024-02-19T18:41:35.654Z <Engine:5:Engine.js:1624> _process_reaction_queue() wake-up! [latest-23338]2024-02-19T18:41:35.655Z <Engine:5:Engine.js:1563> _process_reaction_queue() running task 22989: [Object]{ "tid": 22989, "id": "rule-klten9w9:R", "rule": "rule-klten9w9", "__reaction": [RuleReaction#rule-klten9w9:R], "next_step": 0, "status": 0, "ts": 1708368095651, "parent": --null--, "__resolve": --function--, "__reject": --function--, "__promise": [object Promise] } [latest-23338]2024-02-19T18:41:35.657Z <Engine:NOTICE> Starting reaction Front Cam Motion Detection ON or OFF Old Method<RESET> (rule-klten9w9:R) [latest-23338]2024-02-19T18:41:35.659Z <Engine:5:Engine.js:1701> [Engine]Engine#1 reaction rule-klten9w9:R step 1 perform [Object]{ "entity": "vera>system", "action": "x_vera_sys.runlua", "args": { "lua": "VeraScenes.FrontCamMotionDetectionOff()" } } [latest-23338]2024-02-19T18:41:35.660Z <Engine:5:Engine.js:1577> _process_reaction_queue() task returned, new status 3; task 22989, history 1629572 [latest-23338]2024-02-19T18:41:35.663Z <Engine:5:Engine.js:1624> _process_reaction_queue ending with 1 in queue; none delayed/ready; waiting [latest-23338]2024-02-19T18:41:35.671Z <VeraController:ERR> [VeraController:performOnEntity] action request failed [latest-23338]2024-02-19T18:41:35.672Z <VeraController:CRIT> Error: Request failed: 401 Error [-] Error: Request failed: 401 Error at /home/stuart/reactor/server/lib/Controller.js:886:37 at process.processTicksAndRejections (node:internal/process/task_queues:95:5) [latest-23338]2024-02-19T18:41:35.673Z <Engine:ERR> [Engine]Engine#1 reaction rule-klten9w9:R step 1 perform x_vera_sys.runlua failed: [Error] Request failed: 401 Error [-] [latest-23338]2024-02-19T18:41:35.673Z <Engine:INFO> [Engine]Engine#1 action args: [Object]{ "lua": "VeraScenes.FrontCamMotionDetectionOff()" } [latest-23338]2024-02-19T18:41:35.674Z <Engine:5:Engine.js:1624> _process_reaction_queue() wake-up! [latest-23338]2024-02-19T18:41:35.675Z <Engine:5:Engine.js:1563> _process_reaction_queue() running task 22989: [Object]{ "tid": 22989, "id": "rule-klten9w9:R", "rule": "rule-klten9w9", "__reaction": [RuleReaction#rule-klten9w9:R], "next_step": 2, "status": 1, "ts": 1708368095651, "parent": --null--, "__resolve": --function--, "__reject": --function--, "__promise": [object Promise], "attempts": 0, "history_id": 1629572 } [latest-23338]2024-02-19T18:41:35.676Z <Engine:INFO> Resuming reaction Front Cam Motion Detection ON or OFF Old Method<RESET> (rule-klten9w9:R) from step 2 [latest-23338]2024-02-19T18:41:35.678Z <Engine:5:Engine.js:1753> [Engine]Engine#1 reaction rule-klten9w9:R step 3 notify Telegram with [Object]{ "message": "Front Garden CAM Motion Detection Off", "profile": "default" } [latest-23338]2024-02-19T18:41:35.685Z <Engine:INFO> Front Cam Motion Detection ON or OFF Old Method<RESET> all actions completed. [Was anyone else using this method to have Lua code run on your Vera hub being called via an MSR reaction ? Does it still work for you?
Thanks
Hello all, I am trying to create a timer in home assistant that will run for the amount of seconds left in the day. To do so, my plan is to create an expression in MSR where I subtract the seconds elapsed in the day from 86400. From there I will run a service command to start the timer for the seconds I calculated in the expression. I have tried using the time () function in MSR but cannot figure out a way to just get the time elapsed today and in seconds.
Expressions and LuaXP Functions
-
@toggledbits , I notice that the expression
A = [1,2,3], push(A,A)
does not generate an error (it should, since
push(a,b)
expects a non-object in b), nor does it generate a result. Just limbo. -
This will fall into the categories of "don't do this" and "will not be fixed". The reason is objects (which arrays are) pass by reference so that (in more typical usage)
push(A, 55)
can fulfill its definition: modifying A in place by appending 55 to it. An attempt to fix it would result in having to pass copies of the arguments (pass by value), which means the first argument then is not A, but rather a copy of A, and thenpush()
is modifying the copy in place and not the original array, so the original array never changes, defeating the purpose of the function. -
Forging ahead with more newfangled expressions...
@toggledbits what would you expect from:a=["a","b","c"], b=["d","e"], each val in b: push(a,val)
My money was on:
["a","b","c","d","e"]
but instead I got:
[["a","b","c","d","e"],["a","b","c","d","e"]]
EDIT: Ah, here's the magic sauce:
a=["a","b","c"], b=["d","e"], each val in b: c = push(a,val), c
Result:
["a","b","c","d","e"]
-
@toggledbits , I see the immediately-above situation has been rectified with 21090, so I'll edit accordingly.
I also see that you've instantiated some of the array- and text-handling functions you mentioned earlier, so THANK YOU!
For example:
concat( [1] , [2] )
now yields[1,2]
slice( [1,2,3] , 1 , 2 )
now yields[2,3]
pop( [1,2,3] )
now yields3
push( [1,2,3] , 5 )
now yields[1,2,3,5]
shift( [1,2,3] )
now yields1
unshift( [1,2,3] , 5 )
now yields[5,1,2,3]
// NOTE: currently only permits a single addend; for instance,unshift( [1],2,3,4 )
results in[2,1]
not[2,3,4,1]
! -
This one has me scratching my head a bit:
b = [ ] , each i in a = [ 1 , 2 ] : do push( b , i ) , push( b , i ) done
// yields
[[1,1,2,2],[1,1,2,2]]
// expecting[1,1,2,2]
while this modified version:
b=[],each i in a=[1,2]: do push(b,i) done
// yields
[[1,2],[1,2]]
// expecting[1,2]
-
The result of push is an array.
-
@toggledbits said in Expressions and LuaXP Functions:
The result of push is an array.
But if you
push ( [ ] , 1 )
shouldn't you get
[ 1 ]
? And not[ [ 1 ] , [ 1 ] ]
? -
It depends on what the complete expression is. Remember that the value of
each
is every non-null expression result during the iteration. So an array of arrays is a very possible response. -
@toggledbits Unless I'm mis-reading the way
each
works, I had anticipated the underlying interpretation of:b=[],each i in a=[1,2]: do push(b,i) done
to be as follows:
"Start with an empty array namedb
. For each item in the array nameda
, starting with1
, append that value into arrayb
. First, you would get[ 1 ]
, and the next iteration, using the2
froma
, append that tob
to yield[ 1 , 2 ]
."Any I missing an important piece of the puzzle? (I do recognize that
do
/done
is not necessary here, but I left it in for direct comparison with the expression immediately preceding.)Thanks. Sorry to give you grief over these operations.
-
If you want to see what b looks like at the end, you need to do this:
b=[],each i in a=[1,2]: do push(b,i) done, b
If you don't (which is how you've done it so far), you are seeing the result of
each
, which is an array, and since each expression within theeach
has resulted in an array, the result of thateach
is therefore an array of arrays, and referring to the same array by reference. -
This one seems to evade the purpose of
isnull()
somehow:isnull(1/a) // returns false
whereas
isnull(a) // returns true, as expected
In both cases,
a
is undefined.I also cannot seem to create an expression that results in true for
isNan()
, no matter what I throw at it.Finally, why would
bool(null)
return TRUE? -
null coerces to zero (0) for arithmetic operations, so
1/null
is infinity which is not null.int("abc")
results in NaN which will returnisNaN(int("abc"))==true
bool(null)
should return false and I will fix it.Edit: just to follow up, I've added
Infinity
as a keyword/value to the grammar, with a supportingisInfinity(value)
test function (you can also test viavalue === Infinity
), and added that to the documentation for the next release/build. Andbool(null)
is fixed. FYI,bool()
takes a slightly deeper view of "truthiness" than JavaScript natively: the values (number) 0, null, empty string ("") and NaN are false as in JavaScript, but so additionally in lexpjs/Reactor are string "0", string "no", string "off", and string "false"; any other value of any type is true (including empty arrays and objects). -
MSR RECIPES: Compact object-based message composer
Several of my Rules send out SMTP emails, and I'm always looking for a compact method of composing these outbound messages, using the fewest expressions along with a simple "mail merge" template. Here's a two-variable approach you might consider:
msgBody ► "This is my message." // leave blank if msgBody will be set by other Rules msgTemplate ► tmp = {hdr:"Header here" , msg:msgBody , ftr:"Footer here" , crlf:"\n"}, send = {message:tmp.hdr+tmp.crlf+tmp.msg+tmp.crlf+tmp.ftr} , send.message
I hope this can be adapted to your workflow somehow!
-
Can someone please explain how you would create an array within a Rule that does the following:
(a) begins with an expression that is an empty array;
(b) has another expression containing the .dim_level of a given light;
(c) each time the Rule sets (activates/triggers), the value of (b) is pushed into (a)Working example:
First time light gets turned on, the rule runs. Dim level of 50 is pushed onto end of [ ], making it [50].
Next time light turns on, the rule again runs and pushes the dim level of 75 onto array, making it [50,75].
Etc.I can't quite grok where to put my
push ( )
. In a[Set Variable]
using${{ }}
substitution? In an auxiliary Expression? (I've attempted this approach and can only get one iteration along, meaning the array stops growing after 1 element.) And how does one declare an empty array inside an Expression that will otherwise be modified by the Rule, which normally requires it to be blank?Thanks!
@toggledbits , to put this more succinctly, I find myself unable to implement in MSR the construct you laid out here for creating a time series.
-
SOLUTION: This works for creating a timed data series in MSR...
test1
:= if go=="1" then push( test1 , getEntity( "vera>device_110" ).attributes.dimming.level , 5 ) else test1 endif...BUT...you must first pre-populate thetest1
variable with an empty array[ ]
,SAVE
, then paste in the above definition. Otherwise, MSR will throw a "no such 'push' function on (null)" error! (I call this the "Chicken and the Egg" conundrum, whereas Reactor for Luup doesn't complain about doingpush
to a non-existent array; it just invisibly creates one for you.)In my
set
reaction, I do a[Set Variable] [ go ] = 1
, and in thereset
reaction[Set Variable] [ go ] = 0
, with anInterval
imposed on the Rule's sole Trigger condition, making it goTRUE
for 2 seconds every 60 seconds.NOTE: Interestingly, with
Interval
limited to 3 repeats, the variablego
changes to"1"
only 3 times (expected), yet somehow the array gets fully populated with 5 values (unexpected), so I must not fully understand the leading- and trailing-edge nature ofInterval
as it applies to Expression re-evaluation. I'm sure @toggledbits will elucidate? Or is this truly unexpected behavior?ANSWER: Unchecking "Force re-evaluation of expressions and conditions" in both the
set
andreset
reactions cured the problem of "too many data points". There was my problem. -
@librasun said in Expressions and LuaXP Functions:
you must first pre-populate the test1 variable with an empty array
You don't actually. This is just a bug in lexpjs. I've fixed it in my local copy, will be in the next build. It's just this simple: