A list of openLuup releases including the latest developments…
master – stable, and infrequently updated, development – latest updates and bug fixes, testing – use only when advised!A long while ago (May, 2015) I wrote my 2000-th post on another forum: openLuup - running unmodified plugins on any machine.
Now rehosted at https://community.ezlo.com/t/openluup-running-unmodified-plugins-on-any-machine/187412
Here’s the gist of it:
...I want to work in a more open and stable [Vera] environment...
...All would be solved if Luup was open source and could be run on the plethora of cheap and reliable hardware available today. But it’s not. But we could get something like that effect if we engineered a sufficient subset of Luup to run on such a platform. Could it be done? What would we need?
1. UI
2. scheduler
3. web server
4. Luup compatible API
5. Device and Implementation xml file reader
6. Zwave bridge to Vera
7. runs most plugins without modification
What we wouldn’t need is UPnP.
What have we (nearly) got already?
We have, courtesy of @amg0, the most excellent AltUI: Alternate UI to UI7, and that, I think, is probably the hardest one to do in the above list. Items 2 - 5, and 7, I’ve prototyped, in pure Lua, and posted elsewhere: DataYours on Raspberry Pi, running selected plugins unmodified, including: DataYours, EventWatcher, Netatmo, RBLuaTest, altUI. See screenshot attached.Is it worth the effort? Probably not. Will I pursue this quest? Yes.
openLuup was the result.
Hoping you could tell us a bit about your experiences with ZWaveJS and MQTT.
Akbooer: it would be good if openLuup was added to the awesome mqtt resources list.
How to contribute is described here.
Looks like the GetSolarCoords() doesn't return the correct results. Right Ascension (RA) and
Declination (DEC) look OK. They presumably must be, as I have a light that goes on at sunset at the correct time for years.
Altitude and Azimuth look incorrect. They both have the hour angle in common, so I'm wondering if it's incorrect and hence the sidereal time. Should be able to convert the angle to hours and check it against this clock:
The formula used looks like Compute sidereal time on this page. Might be some mix up between JD2000 that has a 12 hour offset. Could also be some issue with the hour angle.
I'm assuming all Right Ascension (RA) and
Declination (DEC) are degrees plus & minus from north.
Likewise Altitude (ALT) and Azimuth (AZ) are in degrees?
Bit of caution: I haven't looked at this too closely, so may be barking up the wrong tree. It probably doesn't help living near Greenwich.
This site may also be helpful.
PS did you have a look at the link in my last PM?
Set up:
a) Many many many many kms from home: laptop connected to modem router. Router running wireguard client to create a virtual network.
b) Home: modem router running wireguard server. openLuup pi4 connect to router and also a PC and other stuff, etc.
The problem: When accessing charts, AltUI or the openLupp console the web pages are returned OK up to the point where they are truncated and therefore fail to display anything useful.
Note this all works fine over short distances eg around a major city (I tested it) but not seemingly at world wide distances. ie network delays seem to be the issue here? Windows TeamViewer works fine overy the exact same network/wireguard set up. That's how I was able to get the openLuup logs shown below.
Here is any example of openLuup trying to return a chart:
2023-09-04 21:31:20.463 openLuup.io.server:: HTTP:3480 connection from 10.0.0.2 tcp{client}: 0x55aed35038 2023-09-04 21:31:20.464 openLuup.server:: GET /data_request?id=lu_status2&output_format=json&DataVersion=316885191&Timeout=60&MinimumDelay=1500&_=1692128389970 HTTP/1.1 tcp{client}: 0x55ae538348 2023-09-04 21:31:20.465 openLuup.server:: GET /data_request?id=lu_status2&output_format=json&DataVersion=316885191&Timeout=60&MinimumDelay=1500&_=1692129024374 HTTP/1.1 tcp{client}: 0x55addbe1e8 2023-09-04 21:31:20.477 openLuup.server:: GET /data_request?id=lr_render&target={temp_first_floor.w,temp_ground_floor.w,temp_back_wall_of_office.w,temp_inside_roof.w,temp_jps_bedrm_north.w,temp_outside.w}&title=Temperatures&height=750&from=-y&yMin=0&yMax=40 HTTP/1.1 tcp{client}: 0x55aed35038 2023-09-04 21:31:20.478 luup_log:6: DataGraph: drawing mode: connected, draw nulls as: null 2023-09-04 21:31:20.502 luup_log:6: DataGraph: Whisper query: CPU = 23.122 mS for 2016 points 2023-09-04 21:31:20.532 luup_log:6: DataGraph: Whisper query: CPU = 22.952 mS for 2016 points 2023-09-04 21:31:20.561 luup_log:6: DataGraph: Whisper query: CPU = 22.738 mS for 2016 points 2023-09-04 21:31:20.575 luup_log:6: DataGraph: Whisper query: CPU = 9.547 mS for 2016 points 2023-09-04 21:31:20.587 luup_log:6: DataGraph: Whisper query: CPU = 9.569 mS for 2016 points 2023-09-04 21:31:20.598 luup_log:6: DataGraph: Whisper query: CPU = 9.299 mS for 2016 points 2023-09-04 21:31:20.654 luup_log:6: visualization: LineChart(2016x7) 196kB in 51mS 2023-09-04 21:31:20.655 luup_log:6: DataGraph: render: CPU = 51.219 mS for 6x2016=12096 points 2023-09-04 21:31:20.755 openLuup.server:: error 'socket.select() not ready to send tcp{client}: 0x55aed35038' sending 2 bytes to tcp{client}: 0x55aed35038 2023-09-04 21:31:20.855 openLuup.server:: error 'socket.select() not ready to send tcp{client}: 0x55aed35038' sending 6 bytes to tcp{client}: 0x55aed35038 2023-09-04 21:31:21.037 openLuup.server:: error 'socket.select() not ready to send tcp{client}: 0x55aed35038' sending 2 bytes to tcp{client}: 0x55aed35038 2023-09-04 21:31:21.138 openLuup.server:: error 'socket.select() not ready to send tcp{client}: 0x55aed35038' sending 6 bytes to tcp{client}: 0x55aed35038 2023-09-04 21:31:21.332 openLuup.server:: error 'socket.select() not ready to send tcp{client}: 0x55aed35038' sending 2 bytes to tcp{client}: 0x55aed35038 2023-09-04 21:31:21.432 openLuup.server:: error 'socket.select() not ready to send tcp{client}: 0x55aed35038' sending 6 bytes to tcp{client}: 0x55aed35038 2023-09-04 21:31:21.507 openLuup.server:: error 'closed' sending 196367 bytes to tcp{client}: 0x55aed35038 2023-09-04 21:31:21.507 openLuup.server:: ...only 144000 bytes sent 2023-09-04 21:31:21.507 openLuup.server:: error 'closed' sending 2 bytes to tcp{client}: 0x55aed35038 2023-09-04 21:31:21.507 openLuup.server:: ...only 0 bytes sent 2023-09-04 21:31:21.507 openLuup.server:: error 'closed' sending 5 bytes to tcp{client}: 0x55aed35038 2023-09-04 21:31:21.507 openLuup.server:: ...only 0 bytes sent 2023-09-04 21:31:21.507 openLuup.server:: request completed (196367 bytes, 10 chunks, 1030 ms) tcp{client}: 0x55aed35038 2023-09-04 21:31:21.517 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55aed35038 2023-09-04 21:31:22.824 openLuup.io.server:: HTTP:3480 connection from 10.0.0.2 tcp{client}: 0x55aea22c88Re: socket.select() not ready to send
Is there some sort of timeout I change; to see if this can make this work?
Note that openLuup is still running everything flawlessly for ages now, including the more recent addtions of ZigBee stuff. Much appreciated.
Hi @akbooer
Just bringing this over as suggested..
I’ve started to use the console view a lot more, mainly for it’s look and simplicity , but I noticed it does not do any live updates compared to ALTUI, you have to do a full browser reload. Is that by design, or is mine not working?
Also if I want to go strait to the console view, rather than into ALTUI, I recall seeing something abut altering that in the guide by for the life of me I can’t find it. Is it possible to do, if so how would I do that..
You suggested this was something you were looking at ? Also you said You don't need a "full browser reload", just click on the display menu item to refresh the screen. - what do you mean by `display menu?
Very minor issue: was messing about renaming a few rooms and ended up with a room being listed twice. One with the room's contents and the other with no room contents.
It simply turns out one room name had a trailing space. It is possible in both AltUI and the openLuup console to create a room name with a trailing space. Once having done so chaos then ensues, as the rooms are not necessarily treated as different and become difficult to manipulate.
Just need to trim white space off room names. Haven't tested if it's possible to add in leading spaces. That may also be possible.
Hey guys...
Long time... 😉
Since my first day with Vera, I'm using RulesEngine from @vosmont to handle complex rules that will do something based on multiple condition base on "true/false" and also based on time.
Do you think I will be able to do that directly with LUA in openLuup ?
For example..
IF bedroom-motion1 is not detecting motion for 15 minutes
AND
IF bedroom-motion2 is not detecting motion for 15 minutes
AND
IF current-time is between 6am and 11pm
AND
IF binary-light1 is OFF
AND
IF binary-light2 is OFF
THEN
execute LUA code
WAIT 2 minute
execute LUA code
BUT IF any "conditions" failed while in the "THEN" , It need to stop...
I currently have around 60 rules like that 😞
Currently I have a Vera and Hue hub all reliably controlled by openLuup with AltUI, plus any number of plugins. Been working really well for a few years now. However would like to head for a more MQTT based set up. Eliminate the Hue hub and hopefully eliminate Vera by using ZWAVE JS UI. Noting that Zwavejs2mqtt has been renamed to Z-Wave JS UI. Probably also run the stuff using Docker. Just because. Everything would end up on the one computer for easier management. Erhhh that's the hope.
Some of the new Zigbee Aqara stuff is very good and inexpensive plus it fits in with HomeKit. Also the Aqara battery powered stuff looks to have a good battery lifetime: ie suggested up to five years. The battery operated Hue buttons I have; have lasted for ages. Would like to use zigbee2mqtt with a SonOff dongle, which would allow access to the over two and half thousand devices zigbee2mqtt now supports:
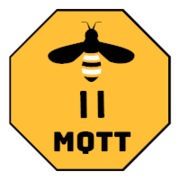
AK has the MQTT stuff working in openLuup. Have played around with it and it works well, as one would expect. Love the UDP to MQTT code.
Shellys are great and also very inexpensive and they spit out & accept MQTT but I would prefer to stay away from WiFi. Not meshed and higher power consumption. Horses for courses.
Now here's the query:
Got about forty or more ZWave twin light switches, plus a few other ZWave bits & pieces such as blind controllers. Then there are the Hue devices on top of that. That's a lot of virtual devices to set up in openLuup. What's an appropriate way to do this?
It seems there is no "auto magic bridge set up". Do I need to use say @therealdb's Virtual Devices plugin that supports MQTT or is there some other approach?
I have to confess I still don't understand the master child approach in that plugin. Seems one light switch would have all the other light switches hanging off it? Helps Vera but not a problem with openLuup - why is that? Suspect AK's good coding beats Vera's?
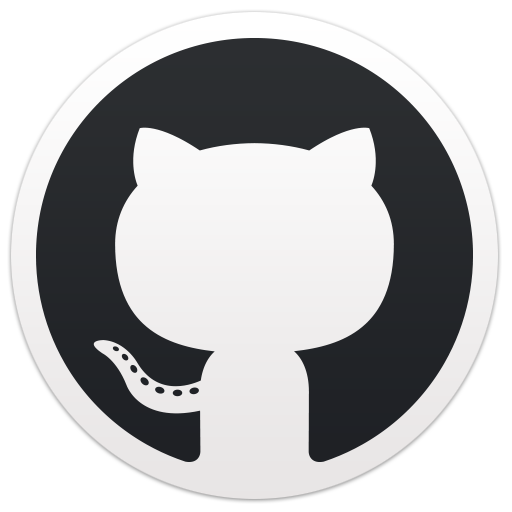
Virtual HTTP Devices plug-in for Vera and openLuup - GitHub - dbochicchio/vera-VirtualDevices: Virtual HTTP Devices plug-in for Vera and openLuup
Setting up manually say 100 virtual devices is a bit much to ask. I had a look at hacking the user_data.json file. Good approach till you see all the UIDs and the individually numbered ControlURL and EventURLs that need to be set up.
I need some way of say of creating about 80 light switches in "No room" or in say the "ZWave upgrade" room. Or say some sort of code that could go through all my existing bridged ZWAVE devices in openLuup and create virtual devices for each one. I caould then use the openLuup console to name them and place them in their rooms:
openLuup_IP_address:3480/console?page=devices_table
At that point I could hack the user_data.json file to insert the MQTT topics fairly easily for each? Plus any other fine tuning needed.
Then the old ZWave stuff could be swapped over to ZWAVE JS UI and all the virtual MQTT devices would be ready to go or am I dreaming? Then delete all the old Vera bridged stuff. I'm not too fussed about scene code and the like, as a I have all my code in one block, that is set up in the openLuup start up.
It seems that with ZWay you can create all the ZWave device by doing some sort of interrogation of ZWay's API? Seems also to be the case with the Shelleys?
So any ideas, suggestions or code snippets are welcome on how to move towards MQTT and in particular ZWAVE JS UI and zigbee2mqtt.
I'm in no hurry as openLuup is performing nicely, with the old Vera handling all my ZWave devices.
Hi
Just wondering if it’s possible when writing plugins to set if the text shown via DisplayLine1/2 can be left, right or centre aligned ?
Bit of an odd one this:
Bare metal install on Debian Bullseye (Intel NUC)
I've noticed when travelling, I connect to my L2TP VPN and I cannot get AltUI to update. I just get 'Waiting Initial Data'
Specifically this is in Chrome:
Version 108.0.5359.94 (Official Build) (x86_64)
In Chrome I can access and control everything via the Openluup console.
In Chrome I can also access and control everything via the Z-Wave expert UI and Z-Wave UI
In Safari I get a more complete view of AltUI but loads of errors along the lines of:
the module or function ALTUI_PluginDisplays.drawBinaryLight does not exist, check your configuration
Homewave on my iOS devices is fine across the same VPN config,
I can ssh into all my servers
Not a huge issue, just curious if anyone has any thoughts of what I might tweak to resolve it?
(FWIW I also access my IMAP and SMTP servers across the same VPN with no issues, as well as remote desktop. Also MS Reactor on the same host as Openluup)
TIA for any thoughts
C
Hi Ak,
Not sure when it started as it took me a while to notice.
I have a function on a luup.call_timer to turn on a switch and then use a luup.call_delay to turn it off a minute later. This is done by the same global function, but on the luup.call_delay i get a message in the log : "luup.call_delay:: unknown global function name: HouseDevice1_PumpCommand"
This is in the init function:
luup.call_timer("HouseDevice1_PumpCommand", 2, "2:15:00", hm_Heating.PumpHealthRunDay, hm_Heating.PumpCMD.HEALTH.."1", true)This is in the function to schedule to off command giving the global function name not found:
luup.call_delay("HouseDevice1_PumpCommand", hm_Heating.PumpHealthOnDuration, hm_Heating.PumpCMD.HEALTH.."0")Is it because I use the "TRUE" parameter that is openLuup specific so the timer does not fire just once?
Running v21.7.25, may be time to update?
Cheers Rene
Hi, I have been trying to install OpenLuup on MacOS but I am failing, so far.
Is there a step-by-step instruction (for MacOS) to follow?
After installing LuaRocks, luasec, luafilesystem and luasocket I then try to run lua5.1 openLuup_install.lua and then get the messages below.
Any ideas and proposals are appreciated.
Regards
Jan
openLuup_install 2019.02.15 @akbooer
lua5.1: openLuup_install.lua:18: module ‘socket.http’ not found:
no field package.preload[‘socket.http’]
no file ‘./socket/http.lua’
no file ‘/usr/local/share/lua/5.1/socket/http.lua’
no file ‘/usr/local/share/lua/5.1/socket/http/init.lua’
no file ‘/usr/local/lib/lua/5.1/socket/http.lua’
no file ‘/usr/local/lib/lua/5.1/socket/http/init.lua’
no file ‘./socket/http.so’
no file ‘/usr/local/lib/lua/5.1/socket/http.so’
no file ‘/usr/local/lib/lua/5.1/loadall.so’
no file ‘./socket.so’
no file ‘/usr/local/lib/lua/5.1/socket.so’
no file ‘/usr/local/lib/lua/5.1/loadall.so’
stack traceback:
[C]: in function ‘require’
openLuup_install.lua:18: in main chunk
Every now and then my openLuup stops responding. This is the LuaUPnP.log when it happens:
2021-08-20 14:59:39.827 :: openLuup LOG ROTATION :: (runtime 11.0 days) 2021-08-20 14:59:39.831 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b6023b8088 2021-08-20 14:59:39.853 openLuup.server:: request completed (49215 bytes, 4 chunks, 22 ms) tcp{client}: 0x55b6023b8088 2021-08-20 14:59:39.854 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b6023b8088 2021-08-20 14:59:40.826 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b600f46ab8 2021-08-20 14:59:40.829 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b600f46ab8 2021-08-20 14:59:40.846 openLuup.server:: request completed (49215 bytes, 4 chunks, 16 ms) tcp{client}: 0x55b600f46ab8 2021-08-20 14:59:40.847 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b600f46ab8 2021-08-20 14:59:41.827 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b600d1ad98 2021-08-20 14:59:41.829 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b600d1ad98 2021-08-20 14:59:41.838 openLuup.server:: request completed (49215 bytes, 4 chunks, 8 ms) tcp{client}: 0x55b600d1ad98 2021-08-20 14:59:41.838 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b600d1ad98 2021-08-20 14:59:42.827 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b6016b9848 2021-08-20 14:59:42.829 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b6016b9848 2021-08-20 14:59:42.847 openLuup.server:: request completed (49215 bytes, 4 chunks, 17 ms) tcp{client}: 0x55b6016b9848 2021-08-20 14:59:42.876 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b6016b9848 2021-08-20 14:59:43.054 luup.watch_callback:: 30003.DS18B20.Temperature called [37]virtualSensorWatchCallback() function: 0x55b600c2fff0 2021-08-20 14:59:43.054 luup.variable_set:: 38.urn:toggledbits-com:serviceId:VirtualSensor1.PreviousRawValue was: 28.3 now: 28.5 #hooks:0 2021-08-20 14:59:43.054 luup.variable_set:: 38.urn:toggledbits-com:serviceId:VirtualSensor1.RawValue was: 28.5 now: 28.4 #hooks:0 2021-08-20 14:59:43.054 luup.variable_set:: 38.urn:upnp-org:serviceId:TemperatureSensor1.CurrentTemperature was: 28.5 now: 28.4 #hooks:0 2021-08-20 14:59:43.054 luup.variable_set:: 38.urn:toggledbits-com:serviceId:VirtualSensor1.PreviousValue was: 28.3 now: 28.5 #hooks:0 2021-08-20 14:59:43.054 luup.variable_set:: 38.urn:toggledbits-com:serviceId:VirtualSensor1.LastUpdate was: 1629464323 now: 1629464383 #hooks:0 2021-08-20 14:59:43.265 openLuup.server:: request completed (3193 bytes, 1 chunks, 5550 ms) tcp{client}: 0x55b60162e488 2021-08-20 14:59:43.267 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b60162e488 2021-08-20 14:59:43.278 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b6023c8458 2021-08-20 14:59:43.278 openLuup.server:: GET /data_request?id=status&DataVersion=516611499&Timeout=15&MinimumDelay=100&output_format=json&_r=1629464383277 HTTP/1.1 tcp{client}: 0x55b6023c8458 2021-08-20 14:59:43.381 openLuup.server:: request completed (1091 bytes, 1 chunks, 102 ms) tcp{client}: 0x55b6023c8458 2021-08-20 14:59:43.381 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b6023c8458 2021-08-20 14:59:43.392 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b6013d6cc8 2021-08-20 14:59:43.393 openLuup.server:: GET /data_request?id=status&DataVersion=516611500&Timeout=15&MinimumDelay=100&output_format=json&_r=1629464383391 HTTP/1.1 tcp{client}: 0x55b6013d6cc8 2021-08-20 14:59:43.496 openLuup.server:: request completed (3196 bytes, 1 chunks, 4981 ms) tcp{client}: 0x55b601a37db8 2021-08-20 14:59:43.826 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601d05a08 2021-08-20 14:59:43.828 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b601d05a08 2021-08-20 14:59:43.844 openLuup.server:: request completed (49215 bytes, 4 chunks, 15 ms) tcp{client}: 0x55b601d05a08 2021-08-20 14:59:43.844 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b601d05a08 2021-08-20 14:59:44.524 openLuup.server:: GET /data_request?id=lu_status2&output_format=json&DataVersion=516611500&Timeout=60&MinimumDelay=1500&_=1629292536029 HTTP/1.1 tcp{client}: 0x55b601a37db8 2021-08-20 14:59:44.828 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b6023a7d18 2021-08-20 14:59:44.831 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b6023a7d18 2021-08-20 14:59:44.844 openLuup.server:: request completed (49215 bytes, 4 chunks, 12 ms) tcp{client}: 0x55b6023a7d18 2021-08-20 14:59:44.844 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b6023a7d18 2021-08-20 14:59:45.514 openLuup.server:: GET /data_request?id=variableget&DeviceNum=0&serviceId=urn:micasaverde-com:serviceId:HomeAutomationGateway1&Variable=Mode&_=1629292536030 HTTP/1.1 tcp{client}: 0x55b601ab8228 2021-08-20 14:59:45.617 openLuup.server:: request completed (1 bytes, 1 chunks, 102 ms) tcp{client}: 0x55b601ab8228 2021-08-20 14:59:45.827 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b600b8de38 2021-08-20 14:59:45.828 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b600b8de38 2021-08-20 14:59:45.834 openLuup.server:: request completed (49215 bytes, 4 chunks, 5 ms) tcp{client}: 0x55b600b8de38 2021-08-20 14:59:45.836 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b600b8de38 2021-08-20 14:59:46.828 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b6018a53f8 2021-08-20 14:59:46.830 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b6018a53f8 2021-08-20 14:59:46.841 openLuup.server:: request completed (49215 bytes, 4 chunks, 11 ms) tcp{client}: 0x55b6018a53f8 2021-08-20 14:59:46.842 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b6018a53f8 2021-08-20 14:59:47.829 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b600d2d938 2021-08-20 14:59:47.832 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b600d2d938 2021-08-20 14:59:47.854 openLuup.server:: request completed (49215 bytes, 4 chunks, 21 ms) tcp{client}: 0x55b600d2d938 2021-08-20 14:59:47.855 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b600d2d938 2021-08-20 14:59:47.962 luup.variable_set:: 10086.urn:upnp-org:serviceId:TemperatureSensor1.CurrentTemperature was: 28.3 now: 28.5 #hooks:0 2021-08-20 14:59:48.370 openLuup.server:: request completed (1359 bytes, 1 chunks, 4977 ms) tcp{client}: 0x55b6013d6cc8 2021-08-20 14:59:48.372 openLuup.server:: request completed (1359 bytes, 1 chunks, 3847 ms) tcp{client}: 0x55b601a37db8 2021-08-20 14:59:48.373 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b6013d6cc8 2021-08-20 14:59:48.384 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601e62df8 2021-08-20 14:59:48.385 openLuup.server:: GET /data_request?id=status&DataVersion=516611502&Timeout=15&MinimumDelay=100&output_format=json&_r=1629464388383 HTTP/1.1 tcp{client}: 0x55b601e62df8 2021-08-20 14:59:48.813 openLuup.server:: GET /data_request?id=lu_status2&output_format=json&DataVersion=516611502&Timeout=60&MinimumDelay=1500&_=1629292536031 HTTP/1.1 tcp{client}: 0x55b601a37db8 2021-08-20 14:59:48.829 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b600c44bf8 2021-08-20 14:59:48.832 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b600c44bf8 2021-08-20 14:59:48.854 openLuup.server:: request completed (49215 bytes, 4 chunks, 21 ms) tcp{client}: 0x55b600c44bf8 2021-08-20 14:59:48.856 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b600c44bf8 2021-08-20 14:59:49.829 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b600e1fea8 2021-08-20 14:59:49.830 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b600e1fea8 2021-08-20 14:59:49.835 openLuup.server:: request completed (49215 bytes, 4 chunks, 5 ms) tcp{client}: 0x55b600e1fea8 2021-08-20 14:59:49.835 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b600e1fea8 2021-08-20 14:59:50.831 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601cfa118 2021-08-20 14:59:50.832 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b601cfa118 2021-08-20 14:59:50.837 openLuup.server:: request completed (49215 bytes, 4 chunks, 4 ms) tcp{client}: 0x55b601cfa118 2021-08-20 14:59:50.838 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b601cfa118 2021-08-20 14:59:51.833 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601462bb8 2021-08-20 14:59:51.835 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b601462bb8 2021-08-20 14:59:51.852 openLuup.server:: request completed (49215 bytes, 4 chunks, 17 ms) tcp{client}: 0x55b601462bb8 2021-08-20 14:59:51.854 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b601462bb8 2021-08-20 14:59:52.834 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b6016b1c98 2021-08-20 14:59:52.836 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b6016b1c98 2021-08-20 14:59:52.847 openLuup.server:: request completed (49215 bytes, 4 chunks, 11 ms) tcp{client}: 0x55b6016b1c98 2021-08-20 14:59:52.848 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b6016b1c98 2021-08-20 14:59:53.193 luup.variable_set:: 10005.urn:micasaverde-com:serviceId:SceneController1.sl_SceneActivated was: 255 now: 255 #hooks:0 2021-08-20 14:59:53.193 luup.variable_set:: 10006.urn:micasaverde-com:serviceId:HaDevice1.sl_TamperAlarm was: 0 now: 0 #hooks:0 2021-08-20 14:59:53.193 luup.variable_set:: 10094.urn:micasaverde-com:serviceId:HaDevice1.sl_BatteryAlarm was: 0 now: 0 #hooks:0 2021-08-20 14:59:53.193 luup.variable_set:: 10096.urn:micasaverde-com:serviceId:HaDevice1.sl_BatteryAlarm was: 10 now: 10 #hooks:0 2021-08-20 14:59:53.193 luup.variable_set:: 10098.urn:micasaverde-com:serviceId:HaDevice1.sl_BatteryAlarm was: 10 now: 10 #hooks:0 2021-08-20 14:59:53.193 luup.variable_set:: 10104.urn:micasaverde-com:serviceId:HaDevice1.sl_BatteryAlarm was: 10 now: 10 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10106.urn:micasaverde-com:serviceId:HaDevice1.sl_BatteryAlarm was: 0 now: 0 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10109.urn:micasaverde-com:serviceId:HaDevice1.sl_BatteryAlarm was: 0 now: 0 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10112.urn:micasaverde-com:serviceId:DoorLock1.sl_UserCode was: UserID="4" UserName="Ella" now: UserID="4" UserName="Ella" #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10112.urn:micasaverde-com:serviceId:DoorLock1.sl_LockButton was: 1 now: 1 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10112.urn:micasaverde-com:serviceId:DoorLock1.sl_PinFailed was: 1 now: 1 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10112.urn:micasaverde-com:serviceId:HaDevice1.sl_BatteryAlarm was: 0 now: 0 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10131.urn:micasaverde-com:serviceId:HaDevice1.sl_TamperAlarm was: 0 now: 0 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10132.urn:micasaverde-com:serviceId:HaDevice1.sl_TamperAlarm was: 0 now: 0 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10133.urn:micasaverde-com:serviceId:HaDevice1.sl_TamperAlarm was: 0 now: 0 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10165.urn:micasaverde-com:serviceId:HaDevice1.sl_Alarm was: SMOKE now: SMOKE #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10165.urn:micasaverde-com:serviceId:HaDevice1.sl_TamperAlarm was: 0 now: 0 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10188.urn:micasaverde-com:serviceId:HaDevice1.sl_TamperAlarm was: 1 now: 1 #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10188.urn:micasaverde-com:serviceId:HaDevice1.sl_Alarm was: TAMPER_ALARM now: TAMPER_ALARM #hooks:0 2021-08-20 14:59:53.194 luup.variable_set:: 10191.urn:micasaverde-com:serviceId:HaDevice1.sl_BatteryAlarm was: 10 now: 10 #hooks:0 2021-08-20 14:59:53.299 openLuup.server:: request completed (5449 bytes, 1 chunks, 4485 ms) tcp{client}: 0x55b601a37db8 2021-08-20 14:59:53.505 openLuup.server:: request completed (5449 bytes, 1 chunks, 5119 ms) tcp{client}: 0x55b601e62df8 2021-08-20 14:59:53.511 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b601e62df8 2021-08-20 14:59:53.513 openLuup.server:: GET /data_request?id=lu_status2&output_format=json&DataVersion=516611523&Timeout=60&MinimumDelay=1500&_=1629292536032 HTTP/1.1 tcp{client}: 0x55b601a37db8 2021-08-20 14:59:53.522 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b600bc7068 2021-08-20 14:59:53.524 openLuup.server:: GET /data_request?id=status&DataVersion=516611523&Timeout=15&MinimumDelay=100&output_format=json&_r=1629464393521 HTTP/1.1 tcp{client}: 0x55b600bc7068 2021-08-20 14:59:53.834 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601b8cbe8 2021-08-20 14:59:53.835 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b601b8cbe8 2021-08-20 14:59:53.840 openLuup.server:: request completed (49215 bytes, 4 chunks, 5 ms) tcp{client}: 0x55b601b8cbe8 2021-08-20 14:59:53.841 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b601b8cbe8 2021-08-20 14:59:54.834 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b6013dacd8 2021-08-20 14:59:54.836 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b6013dacd8 2021-08-20 14:59:54.849 openLuup.server:: request completed (49215 bytes, 4 chunks, 12 ms) tcp{client}: 0x55b6013dacd8 2021-08-20 14:59:54.850 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b6013dacd8 2021-08-20 14:59:55.835 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b602316198 2021-08-20 14:59:55.836 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b602316198 2021-08-20 14:59:55.841 openLuup.server:: request completed (49215 bytes, 4 chunks, 5 ms) tcp{client}: 0x55b602316198 2021-08-20 14:59:55.842 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b602316198 2021-08-20 15:00:10.955 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601547598 2021-08-20 15:00:10.955 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b6016c7cf8 2021-08-20 15:00:10.955 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b600b75158 2021-08-20 15:00:10.955 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b60187d188 2021-08-20 15:00:10.955 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b6011a5bf8 2021-08-20 15:00:10.955 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b60115ecd8 2021-08-20 15:00:10.955 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601018c18 2021-08-20 15:00:10.956 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b60175d068 2021-08-20 15:00:10.956 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b60224ced8 2021-08-20 15:00:10.956 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b6017869d8 2021-08-20 15:00:10.956 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601ab40e8 2021-08-20 15:00:10.956 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601914178 2021-08-20 15:00:10.956 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b60100a228 2021-08-20 15:00:10.956 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b60197a998 2021-08-20 15:00:10.956 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601103238 2021-08-20 15:00:10.956 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b600c62438 2021-08-20 15:00:10.956 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b601eaabf8 2021-08-20 15:00:10.956 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b6017c95b8 2021-08-20 15:00:10.956 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b600d6e508 2021-08-20 15:00:10.956 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b601dd2168 2021-08-20 15:00:10.956 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b60155eb68 2021-08-20 15:00:10.957 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b6016ddcd8 2021-08-20 15:00:10.957 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b602384ca8 2021-08-20 15:00:10.957 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b60230cc78 2021-08-20 15:00:10.957 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b6017e3338 2021-08-20 15:00:10.957 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b600965de8 2021-08-20 15:00:10.957 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b601e23608 2021-08-20 15:00:10.957 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b6018da2f8 2021-08-20 15:00:10.957 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b600c9aa08 2021-08-20 15:00:10.957 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b600bbf0f8 2021-08-20 15:00:10.957 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b601334518 2021-08-20 15:00:10.958 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b60183b5f8 2021-08-20 15:00:10.958 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b6015682a8 2021-08-20 15:00:10.958 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b6018869e8 2021-08-20 15:00:10.958 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b6008839c8 2021-08-20 15:00:10.958 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b60154ed58 2021-08-20 15:00:10.959 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b601a259e8 2021-08-20 15:00:10.959 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b600f617c8 2021-08-20 15:00:10.959 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b6015884c8 2021-08-20 15:00:10.959 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b601c85fe8 2021-08-20 15:00:10.959 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b602275ae8 2021-08-20 15:00:10.959 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b60190ab88 2021-08-20 15:00:10.959 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b601692038 2021-08-20 15:00:10.959 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b601fcea78 2021-08-20 15:00:10.960 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b601ca7f28 2021-08-20 15:00:10.960 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b601fd37f8 2021-08-20 15:00:10.960 openLuup.io.server:: MQTT:1888 connection from 127.0.0.1 tcp{client}: 0x55b6020ec968 2021-08-20 15:00:10.961 openLuup.server:: GET /data_request?id=variableget&DeviceNum=0&serviceId=urn:micasaverde-com:serviceId:HomeAutomationGateway1&Variable=Mode&_=1629292536033 HTTP/1.1 tcp{client}: 0x55b601ab8228 2021-08-20 15:00:10.967 luup_log:0: 32Mb, 3.3%cpu, 11.0days 2021-08-20 15:00:10.972 openLuup.server:: request completed (3361 bytes, 1 chunks, 17459 ms) tcp{client}: 0x55b601a37db8 2021-08-20 15:00:10.973 openLuup.server:: request completed (3361 bytes, 1 chunks, 17449 ms) tcp{client}: 0x55b600bc7068 2021-08-20 15:00:10.990 luup.variable_set:: 10116.urn:upnp-org:serviceId:TemperatureSensor1.CurrentTemperature was: 21.8 now: 21.9 #hooks:0 2021-08-20 15:00:10.990 luup.variable_set:: 10129.urn:micasaverde-com:serviceId:HumiditySensor1.CurrentLevel was: 58.8 now: 58.7 #hooks:0 2021-08-20 15:00:10.991 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600ba45a8 2021-08-20 15:00:10.991 openLuup.mqtt:: closed tcp{client}: 0x55b600ba45a8 2021-08-20 15:00:10.992 openLuup.mqtt:: mqttthing_Klimat kllaren_838dee2e UNSUBSCRIBE from tele/Källartemp/SENSOR tcp{client}: 0x55b600ba45a8 2021-08-20 15:00:10.992 openLuup.mqtt:: ERROR publishing application message for mqtt:tele/Källartemp/SENSOR : closed 2021-08-20 15:00:10.992 openLuup.io.server:: HTTP:3480 connection from 127.0.0.1 tcp{client}: 0x55b601c8c288 2021-08-20 15:00:10.992 openLuup.io.server:: HTTP:3480 connection closed openLuup.server.receive closed tcp{client}: 0x55b600bc7068 2021-08-20 15:00:10.992 openLuup.server:: GET /data_request?id=sdata HTTP/1.1 tcp{client}: 0x55b601547598 2021-08-20 15:00:10.993 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600a62818 2021-08-20 15:00:10.993 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600a62818 2021-08-20 15:00:10.994 openLuup.mqtt:: mqttthing_Uttag garaget_6f4265a1 UNSUBSCRIBE from openLuup/update/10149/SwitchPower1/Status tcp{client}: 0x55b600a62818 2021-08-20 15:00:10.994 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600ba8428 2021-08-20 15:00:10.994 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600ba8428 2021-08-20 15:00:10.994 openLuup.mqtt:: mqttthing_Takflkt hallen_6da387bf UNSUBSCRIBE from tele/TakfläktHall/LWT tcp{client}: 0x55b600ba8428 2021-08-20 15:00:10.994 openLuup.mqtt:: mqttthing_Takflkt hallen_6da387bf UNSUBSCRIBE from stat/TakfläktHall/POWER2 tcp{client}: 0x55b600ba8428 2021-08-20 15:00:10.994 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600e59b88 2021-08-20 15:00:10.994 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600e59b88 2021-08-20 15:00:10.994 openLuup.mqtt:: mqttthing_Klimat sovrummet_0d664e41 UNSUBSCRIBE from openLuup/update/20010/HumiditySensor1/CurrentLevel tcp{client}: 0x55b600e59b88 2021-08-20 15:00:10.994 openLuup.mqtt:: mqttthing_Klimat sovrummet_0d664e41 UNSUBSCRIBE from openLuup/update/20021/TemperatureSensor1/CurrentTemperature tcp{client}: 0x55b600e59b88 2021-08-20 15:00:10.994 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600bd02d8 2021-08-20 15:00:10.994 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600bd02d8 2021-08-20 15:00:10.994 openLuup.mqtt:: mqttthing_Entrdrr_6acda58a UNSUBSCRIBE from openLuup/update/10095/SecuritySensor1/Tripped tcp{client}: 0x55b600bd02d8 2021-08-20 15:00:10.995 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600bb6cf8 2021-08-20 15:00:10.995 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600bb6cf8 2021-08-20 15:00:10.995 openLuup.mqtt:: mqttthing_Temperatur poolen_a589d192 UNSUBSCRIBE from tele/Pooltemp/SENSOR tcp{client}: 0x55b600bb6cf8 2021-08-20 15:00:10.995 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b6009ff868 2021-08-20 15:00:10.995 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b6009ff868 2021-08-20 15:00:10.995 openLuup.mqtt:: mqttthing_Rrelsesensor hallen_cf7c8293 UNSUBSCRIBE from openLuup/update/20013/SecuritySensor1/Tripped tcp{client}: 0x55b6009ff868 2021-08-20 15:00:10.995 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600a23e28 2021-08-20 15:00:10.995 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600a23e28 2021-08-20 15:00:10.995 openLuup.mqtt:: mqttthing_Luftfuktighet badrum_d79a0ffb UNSUBSCRIBE from openLuup/update/10190/HumiditySensor1/CurrentLevel tcp{client}: 0x55b600a23e28 2021-08-20 15:00:10.995 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600bb33b8 2021-08-20 15:00:10.995 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600bb33b8 2021-08-20 15:00:10.995 openLuup.mqtt:: mqttthing_Ljussensor hallen_19569c17 UNSUBSCRIBE from openLuup/update/20006/LightSensor1/CurrentLevel tcp{client}: 0x55b600bb33b8 2021-08-20 15:00:10.995 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600a136b8 2021-08-20 15:00:10.995 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600a136b8 2021-08-20 15:00:10.995 openLuup.mqtt:: mqttthing_Ljussensor trningsrum_ebf319c5 UNSUBSCRIBE from openLuup/update/20004/LightSensor1/CurrentLevel tcp{client}: 0x55b600a136b8 2021-08-20 15:00:10.995 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600babaa8 2021-08-20 15:00:10.995 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600babaa8 2021-08-20 15:00:10.995 openLuup.mqtt:: mqttthing_Solceller watt_e351a23b UNSUBSCRIBE from openLuup/update/10163/LightSensor1/CurrentLevel tcp{client}: 0x55b600babaa8 2021-08-20 15:00:10.995 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b6004a9de8 2021-08-20 15:00:10.995 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b6004a9de8 2021-08-20 15:00:10.995 openLuup.mqtt:: mqttthing_Luftfuktighet dusch_91df9a58 UNSUBSCRIBE from openLuup/update/20008/HumiditySensor1/CurrentLevel tcp{client}: 0x55b6004a9de8 2021-08-20 15:00:10.995 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600b84628 2021-08-20 15:00:10.995 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600b84628 2021-08-20 15:00:10.996 openLuup.mqtt:: mqttthing_Altandrr kk_db6d3edd UNSUBSCRIBE from openLuup/update/10097/SecuritySensor1/Tripped tcp{client}: 0x55b600b84628 2021-08-20 15:00:10.996 openLuup.io.server:: MQTT:1888 connection closed tcp{client}: 0x55b600be6548 2021-08-20 15:00:10.996 openLuup.mqtt:: RECEIVE ERROR: closed tcp{client}: 0x55b600be6548 2021-08-20 15:00:10.996 openLuup.mqtt:: mqttthing_Test datarum_adbe85e6 UNSUBSCRIBE from openLuup/update/20007/Dimming1/LoadLevelTarget tcp{client}: 0x55b600be6548 2021-08-20 15:00:10.996 openLuup.mqtt:: mqttthing_Test datarum_adbe85e6 UNSUBSCRIBE from openLuup/update/20007/SwitchPower1/Status tcp{client}: 0x55b600be6548And then in syslog:
Aug 20 15:00:11 username systemd[1]: openluup.service: Control process exited, code=exited status=7 Aug 20 15:00:11 username systemd[1]: openluup.service: Failed with result 'exit-code'.Wanted to check if was day or night and figured I would use:
local altitude = luup.variable_get(SID.SOLAR, 'ALT', ID.OPEN_LUUP)If altitude is positive it's day, else it's night. However when I read these variables they are incorrect for my locale. The actual values for sunset and midday are correct but do not occur at sunset or midday. I have checked the clock that openLuup uses and it's correct.
Now the time for midday is one hour out and the time for sunset is 13 hours out.
I checked this by getting the UNIX epoch for my location for both sunset and midday and then adding the hours of offset and then called:
local ID = { OPEN_LUUP = 2 } local SID = { OPEN_LUUP = "openLuup", SOLAR = "solar" } --local localTime = my_epoch_for_midday + (3600*1) local localTime = my_epoch_for_sunset + (3600*13) local coords = {Epoch = localTime, '', Latitude = '', Longitude = ''} luup.call_action(SID.SOLAR, "GetSolarCoords", coords, ID.OPEN_LUUP) local altitude = luup.variable_get(SID.SOLAR, 'ALT', ID.OPEN_LUUP) local azimuth = luup.variable_get(SID.SOLAR, 'AZ', ID.OPEN_LUUP) print('New altitude: '..altitude) print('New azimuth: '..azimuth)and I get the right answers.
Not sure what I'm doing wrong. Or perhaps there is some code mix up with UTC versus local time. I checked the documentation but no mention of the solar variables.
If I look at the chart it looks like the whole thing is correct but shifted by my timezone. However I wouldn't expect the timezone to come into play in these calculations.
Feature request: would like to see an openLuup variable that is true for day and false for night. eg IsDay
Feedback / issues with openLuup's built-in MQTT server
Creating a separate thread for anyone who is interested in using the basic (vera) smartphone UI with openLuup
B834C005-6193-4954-95B0-6D020EAEEFDD.jpeg
The actual L_sPhoneUI.lua file needed comes from the ‘ Smartphone Web Interface ’ that’s installed via the mios App Store.
Once you’ve got the file and have uploaded it to openLuup, add the following to your Lua Startup
dofile ("L_sPhoneUI.lua")FYI - all associated js, css and graphic files seem to be hosted at https://download1.mios.com/L_sPhoneUI_layout so not sure how long they will remain available there, but theoretically alternative hosting could be done..
Hi,
I’ve got the L_sPhoneUI.lua file, which is the very basic Vera smartphone UI, and it’s just a do - end code construct. How do I make that work under OpenLuup?
do .. then loads of code here.. endI’ve tried putting into the normal xml/json plugin space but it does seem to get picked up, as none of the handlers are registered?
call_action SendConfig
-
In our last coding with @rafale77 when we work all together to handle zway in openLuup, I asked to be able to send some data directly to the zwave device to change some "parameters" and you made the SendConfig like that
luup.call_action("urn:micasaverde-com:serviceId:HaDevice1","SendConfig",{parameter=6, command=0, size = 0}, ID["D-Bathroom0"])
and now, I would need to be able to do the opposite, "GET" the value from the device instead of SEND
-
It is this function at line 521 of the z-way bridge
SendConfig = function (d,args) local cc = 112 local par,cmd,sz = args.parameter, args.command, args.size or 0 local data = "Set(%s,%s,%s)" data = data: format(par,cmd,sz) local altid = luup.devices[d].id local id, inst = altid: match (NIaltid) Z.zwcommand(id, inst, cc, data) end,
We can add a get function but will have to create a variable to store the return...
GetConfig = function (d,args) local cc = 112 local par = args.parameter local data = "Get(%s)" data = data: format(par) local altid = luup.devices[d].id local id, inst = altid: match (NIaltid) status, response = Z.zwcommand(id, inst, cc, data) luup.variable_set(SRV.HaDevice, "config", response, d) end,
-
Interesting... I did not know that. I submitted a pull request on github though I have not tested it. Take a look.
Edit: I am able to get z-way to send a get parameter command but it doesn't appear to return the correct value. It seems to always be returning 0.
-
Try the updated plugin file below. I can't figure out how to make a luup.call_action return any value other than 0 but... I created 2 new functions:
-GetConfig which will get z-way to poll the parameter value from the device.
-UpdateConfig which gets the value from z-way-server to openLuup and saves it to a device variableThe way to use them is for your example
luup.call_action("urn:micasaverde-com:serviceId:HaDevice1","GetConfig",{parameter=6}, ID["D-Bathroom0"]) luup.call_action("urn:micasaverde-com:serviceId:HaDevice1","UpdateConfig",{parameter=6}, ID["D-Bathroom0"])
Which will create and update a variable called Config(6) which will have the value you are looking for.
-
@rafale77 said in call_action SendConfig:
Interesting... I did not know that.
Yes, indeed. Standard behaviour for
luup.call_action()
as documented here:http://wiki.micasaverde.com/index.php/Luup_Lua_extensions#function:_call_action
The fourth return parameter is a table of named return arguments.
-
@rafale77 said in call_action SendConfig:
Try the updated plugin file below.
A few problems here:
GetConfig()
data2 = data2: format(par)
...this variable is completely undefined, and this line should cause an error.
UpdateConfig()
response = Z.zwcommand(id, inst, cc, data)
this should really be a local:
local response = Z.zwcommand(id, inst, cc, data)
However, I'd prefer an implementation which used the luup.call_action() return parameter. I'll provide a version a bit later.
-
Here's a skeleton for the code that you need:
GetConfig = { run = function() -- do whatever you need to get the status into ZWay_ZWaveCONFIG ZWay_ZWaveCONFIG = 42 end, extra_returns = {Config = function () return ZWay_ZWaveCONFIG end} },
I'm unable to work out from your posted code exactly what you need to do to get the value you want, but just provided a dummy line which sets a global to
42
.Running this from Lua Test on my machine:
local d = 23 local sid = "urn:micasaverde-com:serviceId:HaDevice1" local e,m,j,a = luup.call_action(sid, "GetConfig", {}, d) print(pretty {e,m,j,a})
gives the output:
{0,"",0,{Config = 42}}
-
Thank you. This is what the code should look like:
GetConfig = { run = function() local cc = 112 local par = args.parameter local data = "Get(%s)" data = data: format(par) local data2 = "data[%s].val.value" data2 = data2: format(par) local altid = luup.devices[d].id local id, inst = altid: match (NIaltid) Z.zwcommand(id, inst, cc, data) extra_returns = {Config = function () return Z.zwcommand(id, inst, cc, data) end} },
Note, there might need to be a small delay between the two zwcommand executions to allow time for away to get the parameter from the device which is why I had the idea of splitting out the two actions.
Note that I also modified the zwcommand function for it to return a variable. -
That has a syntax error... you are missing an
end
.Should be:
GetConfig = { run = function() local cc = 112 local par = args.parameter local data = "Get(%s)" data = data: format(par) local data2 = "data[%s].val.value" data2 = data2: format(par) local altid = luup.devices[d].id local id, inst = altid: match (NIaltid) Z.zwcommand(id, inst, cc, data) end, extra_returns = {Config = function () return Z.zwcommand(id, inst, cc, data) end} }
-
another error on my part...
the extra return command should use data2
GetConfig = { run = function() local cc = 112 local par = args.parameter local data = "Get(%s)" data = data: format(par) local data2 = "data[%s].val.value" data2 = data2: format(par) local altid = luup.devices[d].id local id, inst = altid: match (NIaltid) Z.zwcommand(id, inst, cc, data) end, extra_returns = {Config = function () return Z.zwcommand(id, inst, cc, data2) end} }
Edit: don't the variables need to be global to be reused in another function? Do they need to be redefined in the extra_returns function?
-
@rafale77 said in call_action SendConfig:
don't the variables need to be global to be reused in another function?
Yes, that's true. It's why in my original example I used a global for the single return parameter. You could simply do that here by including the second call at the end of the main
GetConfig()
function. -
obviously it is work in progress. The way I implemented it works in spite of the typos but it's not ideal. With AK's help, I think we can get something better.
@akbooer if I run the command as part of the main run command, the return is a function... not a variable as I was expecting. It is pretty strange since I get the variable if I take that return and do a luup.variable_set() with it.
-
I'm playing with the SendConfig a lot since couple of days and I can tell you that I need to put some delay between a couple of SendConfig as something it's not working all the time mixed with some "usual" zwave command!
For example, at night, I turn off a couple of zwave dimmer/switch and sending a couple of SendConfig and the SendConfig are not working 100% of the time!
Maybe zwave stack is handling on/off command differently from "SendConfig" in the queue!